#python code
import cv2
import numpy as np
def mask_setTo():
src = cv2.imread("D:\\projects\\SampleCode\\006939-master\\ch04\\utils\\lenna.bmp", cv2.IMREAD_COLOR)
mask = cv2.imread("D:\\projects\\SampleCode\\006939-master\\ch04\\utils\\mask_smile.bmp", cv2.IMREAD_GRAYSCALE)
if src is None or mask is None:
print("Image load failed!")
return
src[mask > 0] = (0, 255, 255)
cv2.imshow("src", src)
cv2.imshow("mask", mask)
cv2.waitKey(0)
cv2.destroyAllWindows()
def mask_copyTo():
src = cv2.imread("D:\\projects\\SampleCode\\006939-master\\ch04\\utils\\airplane.bmp", cv2.IMREAD_COLOR)
mask = cv2.imread("D:\\projects\\SampleCode\\006939-master\\ch04\\utils\\mask_plane.bmp", cv2.IMREAD_GRAYSCALE)
dst = cv2.imread("D:\\projects\\SampleCode\\006939-master\\ch04\\utils\\field.bmp", cv2.IMREAD_COLOR)
if src is None or mask is None or dst is None:
print("Image load failed!")
return
dst[mask > 0] = src[mask > 0]
cv2.imshow("src", src)
cv2.imshow("dst", dst)
cv2.imshow("mask", mask)
cv2.waitKey(0)
cv2.destroyAllWindows()
def time_inverse():
src = cv2.imread("D:\\projects\\SampleCode\\006939-master\\ch04\\utils\\lenna.bmp", cv2.IMREAD_GRAYSCALE)
if src is None:
print("Image load failed!")
return
dst = cv2.bitwise_not(src)
cv2.imshow("src", src)
cv2.imshow("dst", dst)
cv2.waitKey(0)
cv2.destroyAllWindows()
def useful_func():
img = cv2.imread("D:\\projects\\SampleCode\\006939-master\\ch04\\utils\\lenna.bmp", cv2.IMREAD_GRAYSCALE)
print("Sum:", np.sum(img))
print("Mean:", np.mean(img))
minVal, maxVal, minPos, maxPos = cv2.minMaxLoc(img)
print("minVal:", minVal, "at", minPos)
print("maxVal:", maxVal, "at", maxPos)
src = np.array([-1.0, -0.5, 0.0, 0.5, 1.0], dtype=np.float32)
dst = cv2.normalize(src, None, 0, 255, cv2.NORM_MINMAX, cv2.CV_8UC1)
print("src:", src)
print("dst:", dst)
print("cv2.round(2.5):", round(2.5))
print("cv2.round(2.51):", round(2.51))
print("cv2.round(3.4999):", round(3.4999))
print("cv2.round(3.5):", round(3.5))
def main():
mask_setTo()
mask_copyTo()
time_inverse()
useful_func()
if __name__ == "__main__":
main()
// C++ code
#include "opencv2/opencv.hpp"
#include <iostream>
using namespace cv;
using namespace std;
void mask_setTo();
void mask_copyTo();
void time_inverse();
void useful_func();
int main(void)
{
mask_setTo();
mask_copyTo();
time_inverse();
useful_func();
return 0;
}
void mask_setTo()
{
Mat src = imread("lenna.bmp", IMREAD_COLOR);
Mat mask = imread("mask_smile.bmp", IMREAD_GRAYSCALE);
if (src.empty() || mask.empty()) {
cerr << "Image load failed!" << endl;
return;
}
src.setTo(Scalar(0, 255, 255), mask);
imshow("src", src);
imshow("mask", mask);
waitKey(0);
destroyAllWindows();
}
void mask_copyTo()
{
Mat src = imread("airplane.bmp", IMREAD_COLOR);
Mat mask = imread("mask_plane.bmp", IMREAD_GRAYSCALE);
Mat dst = imread("field.bmp", IMREAD_COLOR);
if (src.empty() || mask.empty() || dst.empty()) {
cerr << "Image load failed!" << endl;
return;
}
src.copyTo(dst, mask);
imshow("src", src);
imshow("dst", dst);
imshow("mask", mask);
waitKey(0);
destroyAllWindows();
}
void time_inverse()
{
Mat src = imread("lenna.bmp", IMREAD_GRAYSCALE);
if (src.empty()) {
cerr << "Image load failed!" << endl;
return;
}
Mat dst(src.rows, src.cols, src.type());
TickMeter tm;
tm.start();
for (int j = 0; j < src.rows; j++) {
for (int i = 0; i < src.cols; i++) {
dst.at<uchar>(j, i) = 255 - src.at<uchar>(j, i);
}
}
tm.stop();
cout << "Image inverse took " << tm.getTimeMilli() << "ms." << endl;
}
void useful_func()
{
Mat img = imread("lenna.bmp", IMREAD_GRAYSCALE);
cout << "Sum: " << (int)sum(img)[0] << endl;
cout << "Mean: " << (int)mean(img)[0] << endl;
double minVal, maxVal;
Point minPos, maxPos;
minMaxLoc(img, &minVal, &maxVal, &minPos, &maxPos);
cout << "minVal: " << minVal << " at " << minPos << endl;
cout << "maxVal: " << maxVal << " at " << maxPos << endl;
Mat src = Mat_<float>({ 1, 5 }, { -1.f, -0.5f, 0.f, 0.5f, 1.f });
Mat dst;
normalize(src, dst, 0, 255, NORM_MINMAX, CV_8UC1);
cout << "src: " << src << endl;
cout << "dst: " << dst << endl;
cout << "cvRound(2.5): " << cvRound(2.5) << endl;
cout << "cvRound(2.51): " << cvRound(2.51) << endl;
cout << "cvRound(3.4999): " << cvRound(3.4999) << endl;
cout << "cvRound(3.5): " << cvRound(3.5) << endl;
}
C++ Code 출처 : OpenCV 4로 배우는 컴퓨터 비전과 머신 러닝 - 황선규 저
Sum: 32518590
Mean: 124.04857635498047
minVal: 25.0 at (508, 71)
maxVal: 245.0 at (116, 273)
src: [-1. -0.5 0. 0.5 1. ]
dst: [[ 0]
[ 64]
[128]
[191]
[255]]
cv2.round(2.5): 2
cv2.round(2.51): 3
cv2.round(3.4999): 3
cv2.round(3.5): 4
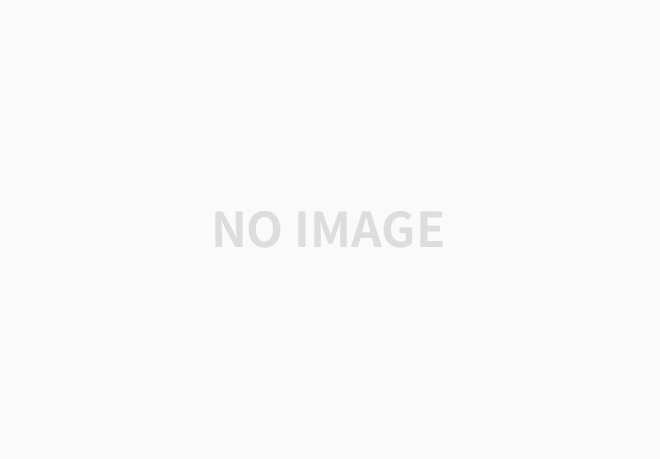

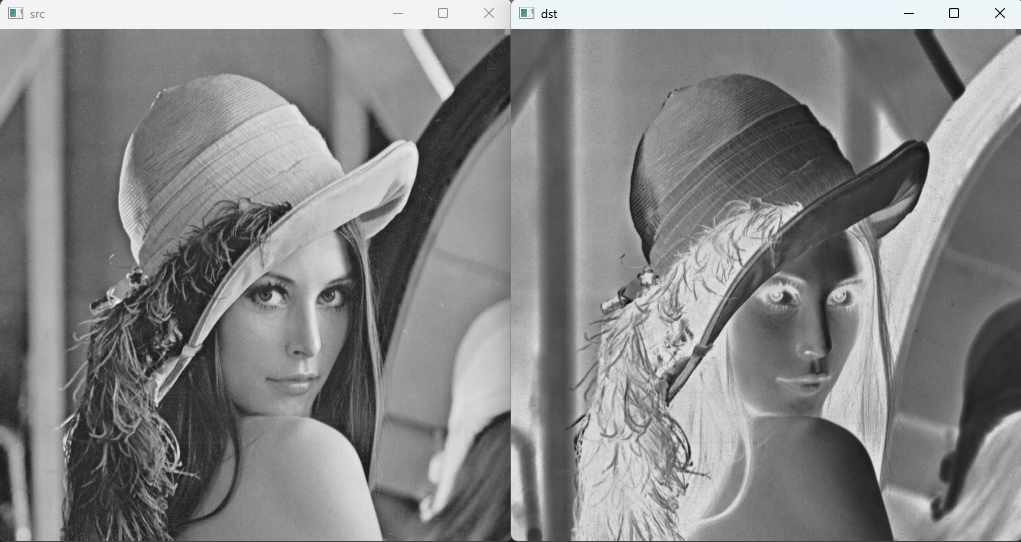
'OpenCV' 카테고리의 다른 글
ch05 brightness (0) | 2024.05.19 |
---|---|
ch04 video (0) | 2024.05.19 |
ch04 trackbar (0) | 2024.05.19 |
ch04 trackbar (0) | 2024.05.19 |
ch04 storage (0) | 2024.05.19 |