#python
import cv2
import numpy as np
def set_label(img, pts, label):
rc = cv2.boundingRect(pts)
cv2.rectangle(img, rc, (0, 0, 255), 1)
cv2.putText(img, label, rc[:2], cv2.FONT_HERSHEY_PLAIN, 1, (0, 0, 255))
def main():
img = cv2.imread("D:\\projects\\SampleCode\\006939-master\\ch12\\polygon\\polygon.bmp", cv2.IMREAD_COLOR)
if img is None:
print("Image load failed!")
return -1
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
_, bin = cv2.threshold(gray, 200, 255, cv2.THRESH_BINARY_INV | cv2.THRESH_OTSU)
contours, _ = cv2.findContours(bin, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_NONE)
for pts in contours:
if cv2.contourArea(pts) < 400:
continue
approx = cv2.approxPolyDP(pts, cv2.arcLength(pts, True) * 0.02, True)
vtc = len(approx)
if vtc == 3:
set_label(img, pts, "TRI")
elif vtc == 4:
set_label(img, pts, "RECT")
else:
length = cv2.arcLength(pts, True)
area = cv2.contourArea(pts)
ratio = 4.0 * np.pi * area / (length * length)
if ratio > 0.85:
set_label(img, pts, "CIR")
cv2.imshow("img", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
if __name__ == "__main__":
main()
// C++
#include "opencv2/opencv.hpp"
#include <iostream>
using namespace cv;
using namespace std;
void setLabel(Mat& img, const vector<Point>& pts, const String& label)
{
Rect rc = boundingRect(pts);
rectangle(img, rc, Scalar(0, 0, 255), 1);
putText(img, label, rc.tl(), FONT_HERSHEY_PLAIN, 1, Scalar(0, 0, 255));
}
int main(int argc, char* argv[])
{
Mat img = imread("polygon.bmp", IMREAD_COLOR);
if (img.empty()) {
cerr << "Image load failed!" << endl;
return -1;
}
Mat gray;
cvtColor(img, gray, COLOR_BGR2GRAY);
Mat bin;
threshold(gray, bin, 200, 255, THRESH_BINARY_INV | THRESH_OTSU);
vector<vector<Point>> contours;
findContours(bin, contours, RETR_EXTERNAL, CHAIN_APPROX_NONE);
for (vector<Point> pts : contours) {
if (contourArea(pts) < 400)
continue;
vector<Point> approx;
approxPolyDP(pts, approx, arcLength(pts, true)*0.02, true);
int vtc = (int)approx.size();
if (vtc == 3) {
setLabel(img, pts, "TRI");
} else if (vtc == 4) {
setLabel(img, pts, "RECT");
} else {
double len = arcLength(pts, true);
double area = contourArea(pts);
double ratio = 4. * CV_PI * area / (len * len);
if (ratio > 0.85) {
setLabel(img, pts, "CIR");
}
}
}
imshow("img", img);
waitKey(0);
return 0;
}
C++ Code 출처 : OpenCV 4로 배우는 컴퓨터 비전과 머신 러닝 - 황선규 저
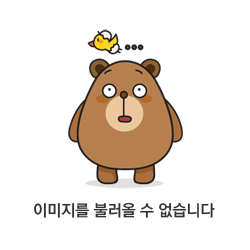
'OpenCV' 카테고리의 다른 글
ch13 hog (0) | 2024.05.21 |
---|---|
ch13 cascade (0) | 2024.05.21 |
ch12 labeling (0) | 2024.05.21 |
ch12 findcts (0) | 2024.05.21 |
ch11 threshold (0) | 2024.05.21 |