#python
import cv2
import sys
def main(argv):
if len(argv) < 3:
print("Usage: python stitching.py <image_file1> <image_file2> [<image_file3> ...]")
return -1
imgs = []
for filename in argv[1:]:
img = cv2.imread(filename)
if img is None:
print("Image load failed!")
return -1
imgs.append(img)
stitcher = cv2.Stitcher.create()
status, dst = stitcher.stitch(imgs)
if status != cv2.Stitcher_OK:
print("Error on stitching!")
return -1
cv2.imwrite("result.jpg", dst)
cv2.imshow("dst", dst)
cv2.waitKey(0)
cv2.destroyAllWindows()
return 0
if __name__ == "__main__":
sys.exit(main(sys.argv))
// C++
#include "opencv2/opencv.hpp"
#include <iostream>
using namespace cv;
using namespace std;
int main(int argc, char* argv[])
{
if (argc < 3) {
cerr << "Usage: stitching.exe <image_file1> <image_file2> [<image_file3> ...]" << endl;
return -1;
}
vector<Mat> imgs;
for (int i = 1; i < argc; i++) {
Mat img = imread(argv[i]);
if (img.empty()) {
cerr << "Image load failed!" << endl;
return -1;
}
imgs.push_back(img);
}
Ptr<Stitcher> stitcher = Stitcher::create();
Mat dst;
Stitcher::Status status = stitcher->stitch(imgs, dst);
if (status != Stitcher::Status::OK) {
cerr << "Error on stitching!" << endl;
return -1;
}
imwrite("result.jpg", dst);
imshow("dst", dst);
waitKey();
return 0;
}
C++ Code 출처 : OpenCV 4로 배우는 컴퓨터 비전과 머신 러닝 - 황선규 저
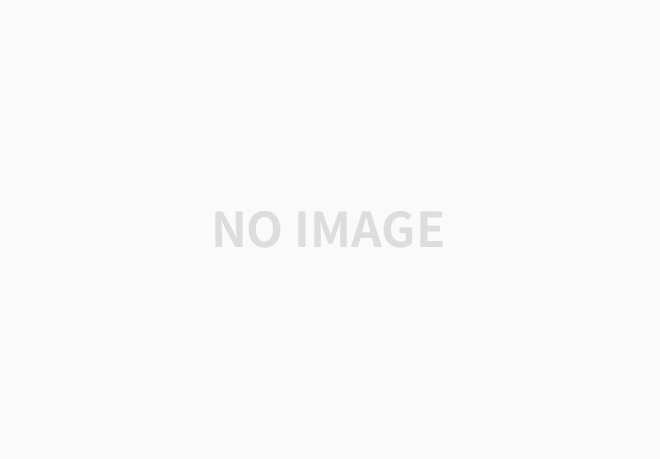
'OpenCV' 카테고리의 다른 글
ch14 matching (0) | 2024.05.22 |
---|---|
ch14 keypoints (0) | 2024.05.22 |
ch14 corners (0) | 2024.05.22 |
ch13 template (0) | 2024.05.21 |
ch13 QRCode (0) | 2024.05.21 |