import cv2
import numpy as np
def MatOp1():
img1 = np.empty((0, 0), dtype=np.uint8) # empty matrix
img2 = np.zeros((480, 640), dtype=np.uint8) # unsigned char, 1-channel
img3 = np.zeros((480, 640, 3), dtype=np.uint8) # unsigned char, 3-channels
img4 = np.zeros((480, 640, 3), dtype=np.uint8) # Size(width, height)
img5 = np.full((480, 640), 128, dtype=np.uint8) # initial values, 128
img6 = np.full((480, 640, 3), (0, 0, 255), dtype=np.uint8) # initial values, red
mat1 = np.zeros((3, 3), dtype=np.int32) # 0's matrix
mat2 = np.ones((3, 3), dtype=np.float32) # 1's matrix
mat3 = np.eye(3, 3, dtype=np.float32) # identity matrix
data = np.array([1, 2, 3, 4, 5, 6], dtype=np.float32).reshape(2, 3)
mat4 = data.copy()
mat5 = np.array([[1, 2, 3], [4, 5, 6]], dtype=np.float32)
mat6 = np.array([[1, 2, 3], [4, 5, 6]], dtype=np.uint8)
mat4 = np.zeros((256, 256, 3), dtype=np.uint8) # uchar, 3-channels
mat5 = np.zeros((4, 4), dtype=np.float32) # float, 1-channel
mat4[:, :] = (255, 0, 0)
mat5[:, :] = 1.0
def MatOp2():
img1 = cv2.imread("D:\\projects\\SampleCode\\006939-master\\ch03\\MatOp\\dog.bmp")
img2 = img1
img3 = img1
img4 = img1.copy()
img5 = img1.copy()
img1[:, :] = (0, 255, 255) # yellow
cv2.imshow("img1", img1)
cv2.imshow("img2", img2)
cv2.imshow("img3", img3)
cv2.imshow("img4", img4)
cv2.imshow("img5", img5)
cv2.waitKey()
cv2.destroyAllWindows()
def MatOp3():
img1 = cv2.imread("D:\\projects\\SampleCode\\006939-master\\ch03\\MatOp\\cat.bmp")
if img1 is None:
print("Image load failed!")
return
img2 = img1[120:360, 220:560]
img3 = img1[120:360, 220:560].copy()
img2[:] = ~img2
cv2.imshow("img1", img1)
cv2.imshow("img2", img2)
cv2.imshow("img3", img3)
cv2.waitKey()
cv2.destroyAllWindows()
def MatOp4():
mat1 = np.zeros((3, 4), dtype=np.uint8)
for j in range(mat1.shape[0]):
for i in range(mat1.shape[1]):
mat1[j, i] += 1
for j in range(mat1.shape[0]):
p = mat1[j]
for i in range(mat1.shape[1]):
p[i] += 1
mat1 = mat1 + 1
print("mat1:\n", mat1)
def MatOp5():
img1 = cv2.imread("D:\\projects\\SampleCode\\006939-master\\ch03\\MatOp\\lenna.bmp")
print("Width:", img1.shape[1])
print("Height:", img1.shape[0])
print("Channels:", img1.shape[2])
if img1.ndim == 2:
print("img1 is a grayscale image.")
elif img1.ndim == 3:
print("img1 is a truecolor image.")
data = np.array([2.0, 1.414, 3.0, 1.732], dtype=np.float32).reshape(2, 2)
mat1 = data
print("mat1:\n", mat1)
def MatOp6():
data = np.array([1, 1, 2, 3], dtype=np.float32).reshape(2, 2)
mat1 = data
print("mat1:\n", mat1)
mat2 = np.linalg.inv(mat1)
print("mat2:\n", mat2)
print("mat1.t():\n", mat1.T)
print("mat1 + 3:\n", mat1 + 3)
print("mat1 + mat2:\n", mat1 + mat2)
print("mat1 * mat2:\n", np.matmul(mat1, mat2))
def MatOp7():
img1 = cv2.imread("D:\\projects\\SampleCode\\006939-master\\ch03\\MatOp\\lenna.bmp", cv2.IMREAD_GRAYSCALE)
img1f = img1.astype(np.float32)
data1 = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12], dtype=np.uint8).reshape(3, 4)
mat1 = data1
mat2 = mat1.reshape(1, -1)
print("mat1:\n", mat1)
print("mat2:\n", mat2)
mat1 = np.resize(mat1, (5, mat1.shape[1]))
print("mat1:\n", mat1)
mat3 = np.ones((1, 4), dtype=np.uint8) * 255
mat1 = np.vstack([mat1, mat3])
print("mat1:\n", mat1)
if __name__ == "__main__":
MatOp1()
MatOp2()
MatOp3()
MatOp4()
MatOp5()
MatOp6()
MatOp7()
// C++ code
#include "opencv2/opencv.hpp"
#include <iostream>
using namespace cv;
using namespace std;
void MatOp1();
void MatOp2();
void MatOp3();
void MatOp4();
void MatOp5();
void MatOp6();
void MatOp7();
int main()
{
MatOp1();
MatOp2();
MatOp3();
MatOp4();
MatOp5();
MatOp6();
MatOp7();
return 0;
}
void MatOp1()
{
Mat img1; // empty matrix
Mat img2(480, 640, CV_8UC1); // unsigned char, 1-channel
Mat img3(480, 640, CV_8UC3); // unsigned char, 3-channels
Mat img4(Size(640, 480), CV_8UC3); // Size(width, height)
Mat img5(480, 640, CV_8UC1, Scalar(128)); // initial values, 128
Mat img6(480, 640, CV_8UC3, Scalar(0, 0, 255)); // initial values, red
Mat mat1 = Mat::zeros(3, 3, CV_32SC1); // 0's matrix
Mat mat2 = Mat::ones(3, 3, CV_32FC1); // 1's matrix
Mat mat3 = Mat::eye(3, 3, CV_32FC1); // identity matrix
float data[] = { 1, 2, 3, 4, 5, 6 };
Mat mat4(2, 3, CV_32FC1, data);
Mat mat5 = (Mat_<float>(2, 3) << 1, 2, 3, 4, 5, 6);
Mat mat6 = Mat_<uchar>({2, 3}, { 1, 2, 3, 4, 5, 6 });
mat4.create(256, 256, CV_8UC3); // uchar, 3-channels
mat5.create(4, 4, CV_32FC1); // float, 1-channel
mat4 = Scalar(255, 0, 0);
mat5.setTo(1.f);
}
void MatOp2()
{
Mat img1 = imread("dog.bmp");
Mat img2 = img1;
Mat img3;
img3 = img1;
Mat img4 = img1.clone();
Mat img5;
img1.copyTo(img5);
img1.setTo(Scalar(0, 255, 255)); // yellow
imshow("img1", img1);
imshow("img2", img2);
imshow("img3", img3);
imshow("img4", img4);
imshow("img5", img5);
waitKey();
destroyAllWindows();
}
void MatOp3()
{
Mat img1 = imread("cat.bmp");
if (img1.empty()) {
cerr << "Image load failed!" << endl;
return;
}
Mat img2 = img1(Rect(220, 120, 340, 240));
Mat img3 = img1(Rect(220, 120, 340, 240)).clone();
img2 = ~img2;
imshow("img1", img1);
imshow("img2", img2);
imshow("img3", img3);
waitKey();
destroyAllWindows();
}
void MatOp4()
{
Mat mat1 = Mat::zeros(3, 4, CV_8UC1);
for (int j = 0; j < mat1.rows; j++) {
for (int i = 0; i < mat1.cols; i++) {
mat1.at<uchar>(j, i)++;
}
}
for (int j = 0; j < mat1.rows; j++) {
uchar* p = mat1.ptr<uchar>(j);
for (int i = 0; i < mat1.cols; i++) {
p[i]++;
}
}
for (MatIterator_<uchar> it = mat1.begin<uchar>(); it != mat1.end<uchar>(); ++it) {
(*it)++;
}
cout << "mat1:\n" << mat1 << endl;
}
void MatOp5()
{
Mat img1 = imread("lenna.bmp");
cout << "Width: " << img1.cols << endl;
cout << "Height: " << img1.rows << endl;
cout << "Channels: " << img1.channels() << endl;
if (img1.type() == CV_8UC1)
cout << "img1 is a grayscale image." << endl;
else if (img1.type() == CV_8UC3)
cout << "img1 is a truecolor image." << endl;
float data[] = { 2.f, 1.414f, 3.f, 1.732f };
Mat mat1(2, 2, CV_32FC1, data);
cout << "mat1:\n" << mat1 << endl;
}
void MatOp6()
{
float data[] = { 1, 1, 2, 3 };
Mat mat1(2, 2, CV_32FC1, data);
cout << "mat1:\n" << mat1 << endl;
Mat mat2 = mat1.inv();
cout << "mat2:\n" << mat2 << endl;
cout << "mat1.t():\n" << mat1.t() << endl;
cout << "mat1 + 3:\n" << mat1 + 3 << endl;
cout << "mat1 + mat2:\n" << mat1 + mat2 << endl;
cout << "mat1 * mat2:\n" << mat1 * mat2 << endl;
}
void MatOp7()
{
Mat img1 = imread("lenna.bmp", IMREAD_GRAYSCALE);
Mat img1f;
img1.convertTo(img1f, CV_32FC1);
uchar data1[] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12 };
Mat mat1(3, 4, CV_8UC1, data1);
Mat mat2 = mat1.reshape(0, 1);
cout << "mat1:\n" << mat1 << endl;
cout << "mat2:\n" << mat2 << endl;
mat1.resize(5, 100);
cout << "mat1:\n" << mat1 << endl;
Mat mat3 = Mat::ones(1, 4, CV_8UC1) * 255;
mat1.push_back(mat3);
cout << "mat1:\n" << mat1 << endl;
}
C++ Code 출처 : OpenCV 4로 배우는 컴퓨터 비전과 머신 러닝 - 황선규 저
mat1:
[[3 3 3 3]
[3 3 3 3]
[3 3 3 3]]
Width: 512
Height: 512
Channels: 3
img1 is a truecolor image.
mat1:
[[2. 1.414]
[3. 1.732]]
mat1:
[[1. 1.]
[2. 3.]]
mat2:
[[ 3. -1.]
[-2. 1.]]
mat1.t():
[[1. 2.]
[1. 3.]]
mat1 + 3:
[[4. 4.]
[5. 6.]]
mat1 + mat2:
[[4. 0.]
[0. 4.]]
mat1 * mat2:
[[1. 0.]
[0. 1.]]
mat1:
[[ 1 2 3 4]
[ 5 6 7 8]
[ 9 10 11 12]]
mat2:
[[ 1 2 3 4 5 6 7 8 9 10 11 12]]
mat1:
[[ 1 2 3 4]
[ 5 6 7 8]
[ 9 10 11 12]
[ 1 2 3 4]
[ 5 6 7 8]]
mat1:
[[ 1 2 3 4]
[ 5 6 7 8]
[ 9 10 11 12]
[ 1 2 3 4]
[ 5 6 7 8]
[255 255 255 255]]
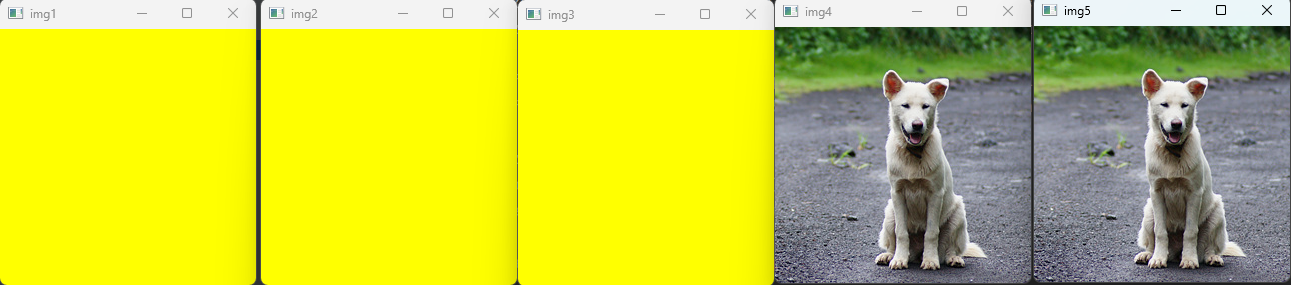
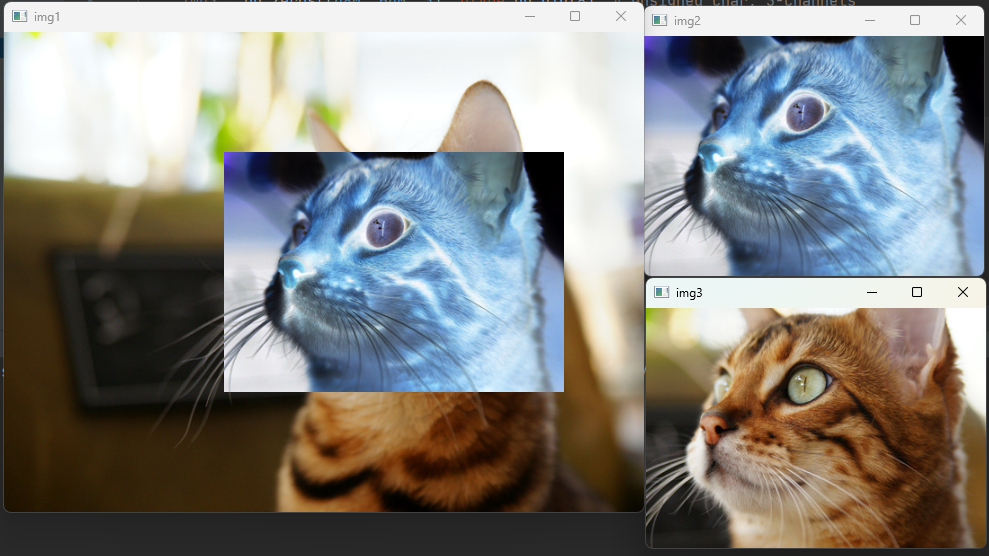
'OpenCV' 카테고리의 다른 글
ch04 drawing (0) | 2024.05.19 |
---|---|
ch03 ScalarOp (0) | 2024.05.19 |
ch03 InputArrayOp (0) | 2024.05.19 |
ch03 BasicOp (0) | 2024.05.19 |
ch02 Hello OpenCV (0) | 2024.05.19 |