#python
import cv2
import numpy as np
# Load the image
src = cv2.imread("D:\\projects\\SampleCode\\006939-master\\ch10\\coloreq\\pepper.bmp", cv2.IMREAD_COLOR)
# Check if the image is loaded successfully
if src is None:
print("Image load failed!")
exit()
# Convert the image to YCrCb color space
src_ycrcb = cv2.cvtColor(src, cv2.COLOR_BGR2YCrCb)
# Split the YCrCb image into its planes
ycrcb_planes = cv2.split(src_ycrcb)
# Apply histogram equalization to the Y channel
cv2.equalizeHist(ycrcb_planes[0], ycrcb_planes[0])
# Merge the planes back into an image
dst_ycrcb = cv2.merge(ycrcb_planes)
# Convert the image back to BGR color space
dst = cv2.cvtColor(dst_ycrcb, cv2.COLOR_YCrCb2BGR)
# Display the original and processed images
cv2.imshow("src", src)
cv2.imshow("dst", dst)
cv2.waitKey(0)
cv2.destroyAllWindows()
// C++
#include "opencv2/opencv.hpp"
#include <iostream>
using namespace cv;
using namespace std;
int main(void)
{
Mat src = imread("pepper.bmp", IMREAD_COLOR);
if (src.empty()) {
cerr << "Image load failed!" << endl;
return -1;
}
Mat src_ycrcb;
cvtColor(src, src_ycrcb, COLOR_BGR2YCrCb);
vector<Mat> ycrcb_planes;
split(src_ycrcb, ycrcb_planes);
equalizeHist(ycrcb_planes[0], ycrcb_planes[0]); // Y channel
Mat dst_ycrcb;
merge(ycrcb_planes, dst_ycrcb);
Mat dst;
cvtColor(dst_ycrcb, dst, COLOR_YCrCb2BGR);
imshow("src", src);
imshow("dst", dst);
waitKey(0);
return 0;
}
C++ Code 출처 : OpenCV 4로 배우는 컴퓨터 비전과 머신 러닝 - 황선규 저
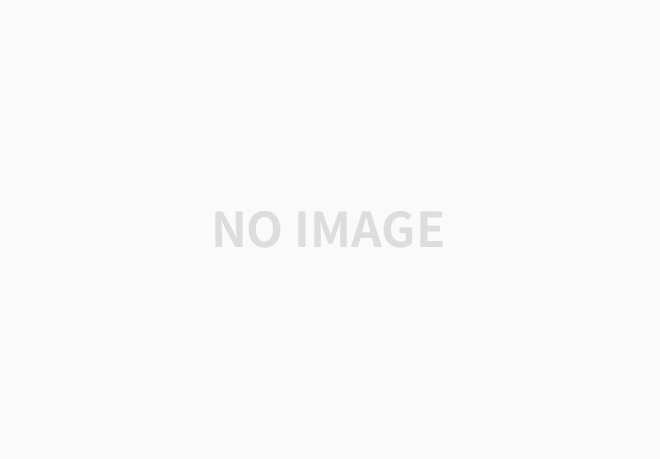
'OpenCV' 카테고리의 다른 글
ch10 inrange (0) | 2024.05.21 |
---|---|
ch10 colorOp (0) | 2024.05.21 |
ch10 backproj (0) | 2024.05.21 |
ch09 hough (0) | 2024.05.20 |
ch09 edges (0) | 2024.05.20 |